Electron Setup
Send push notifications to your Electron app with Pushy.
At any time, you may refer to our Electron demo implementation file for a working demo.
Create an App
Sign up for Pushy Trial to get started, or log in to your existing account
Note: No credit card needed. Upgrade to the Pro plan when you're ready by visiting the Billing page.
Create an app and fill in the Web Push Hostname with your company domain name:
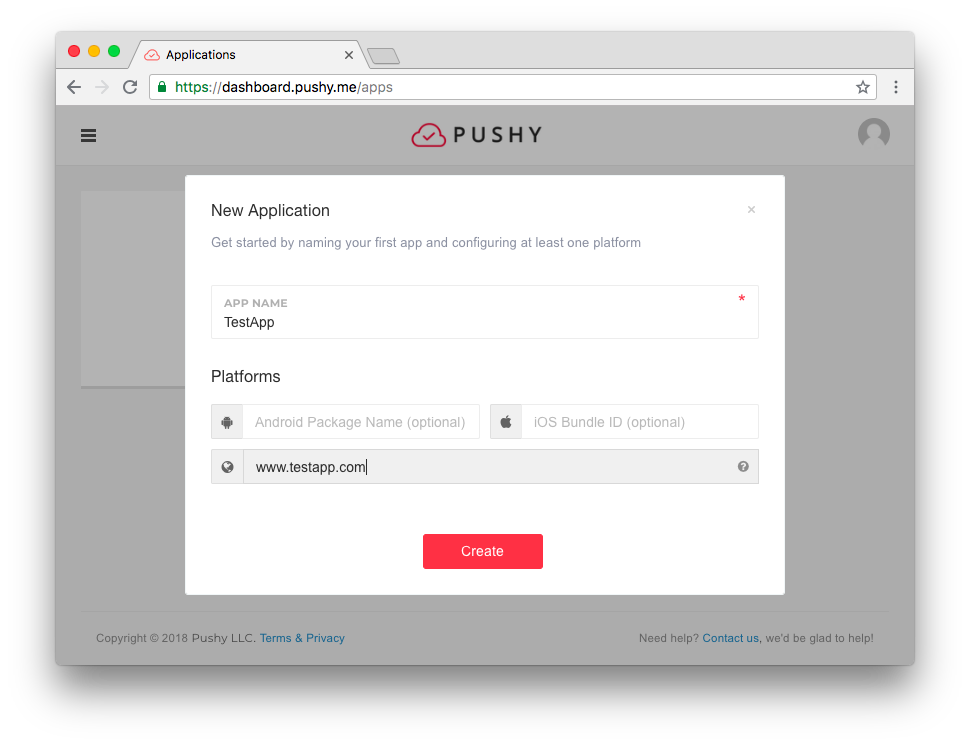
Note: If you have already created an app in the Pushy Dashboard for another platform, simply configure your existing dashboard app with your Web Push Hostname with your company domain name in the App Settings tab and proceed to the next step.
If you're targeting other platforms, please fill in their respective app identifier fields.
Click Create and proceed to the next step.
Install the SDK
Run the following command in the root directory of your project to install the pushy-electron package from npm:
npm install pushy-electron --save
Modify Electron App (ipcMain)
Require the package in your ipcMain
(Node-context) file like so:
const Pushy = require('pushy-electron');
Invoke Pushy.listen()
in did-finish-load()
to initialize the SDK:
// On window load listener
win.webContents.on('did-finish-load', () => {
// Initialize Pushy
Pushy.listen();
});
Register Devices
Users need to be uniquely identified to receive push notifications.
Every user is assigned a unique device token that you can use to push it at any given time. Once the user has been assigned a device token, it should be stored in your application's backend database.
Invoke Pushy.register()
in did-finish-load()
to register devices for notifications:
// Register device for push notifications
Pushy.register({ appId: 'YOUR_APP_ID' }).then((deviceToken) => {
// Display an alert with device token
Pushy.alert(win, 'Pushy device token: ' + deviceToken);
}).catch((err) => {
// Display error dialog
Pushy.alert(win, 'Pushy registration error: ' + err.message);
});
Note: Please make sure to replace YOUR_APP_ID
with your Pushy App ID in the Pushy Dashboard -> Click your app -> App Settings -> App ID.
Listen for Notifications
Invoke Pushy.setNotificationListener((data) => {})
in did-finish-load()
to handle incoming push notifications:
// Listen for push notifications
Pushy.setNotificationListener((data) => {
// Display an alert with the "message" payload value
Pushy.alert(win, 'Received notification: ' + data.message);
});
Feel free to modify this sample code to suit your own needs.
Note: You may currently only register one notification listener at a time for your app.
Parse Notification Data
Any payload data that you send with your push notifications is made available to your app via the data
parameter of your notification listener.
If you were to send a push notification with the following payload:
{"id": 1, "success": true, "message": "Hello World"}
Then you'd be able to retrieve each value from within your notification listener callback like so:
let id = data.id; // number
let success = data.success; // bool
let message = data.message; // string
Note: Unlike GCM / FCM, we do not stringify your payload data, except if you supply JSON objects or arrays.
Subscribe to Topics
Optionally subscribe the user to one or more topics to target multiple users with a shared interest when sending notifications.
Depending on your app's notification criteria, you may be able to leverage topics to simply the process of sending the same notification to multiple users. If your app only sends personalized notifications, skip this step and simply target individual users by their unique device tokens.
Add the following code to subscribe the device to a Pub/Sub topic:
// Make sure the user is registered
if (Pushy.isRegistered()) {
// Subscribe the user to a topic
Pushy.subscribe('news').then(() => {
// Subscribe successful
Pushy.alert(win, 'Subscribed to topic successfully');
}).catch((err) => {
// Notify user of failure
Pushy.alert(win, 'Pushy subscribe error: ' + err.message);
});
}
Note: Replace news
with your own case-sensitive topic name that matches the following regular expression: [a-zA-Z0-9-_.]{1,100}
.
You can then notify multiple users subscribed to a certain topic by specifying the topic name (prefixed with /topics/
) as the to
parameter in the Send Notifications API.
Send Test Notification
Run your Electron app, copy and paste the generated device token from the console, and select your app to send a test push notification:
Note: You can specify a topic instead of a device token (i.e. /topics/news
). Also, if your app is not automatically detected, please manually copy the Secret API Key from the Dashboard and paste it into the form.
Did you receive the notification? If not, reach out, we'll be glad to help.
Congratulations on implementing Pushy in your Electron app!
To start sending push notifications to your users, start persisting device tokens in your backend, and invoke the Send Notifications API when you want to send a notification. Follow our step-by-step guide: