React Native Setup
Send push notifications to your React Native and Expo apps with Pushy.
At any time, you may refer to our React Native and Expo demo projects for a working demo.
Create an App
Sign up for Pushy Trial to get started, or log in to your existing account
Note: No credit card needed. Upgrade to the Pro plan when you're ready by visiting the Billing page.
Create an app and fill in the Android Package Name & iOS Bundle ID:
Please ensure the Android Package Name & iOS Bundle ID you enter precisely match the ones configured in your project files, as they are used to automatically link your client apps with the Pushy Dashboard app having the same Android Package Name & iOS Bundle ID.
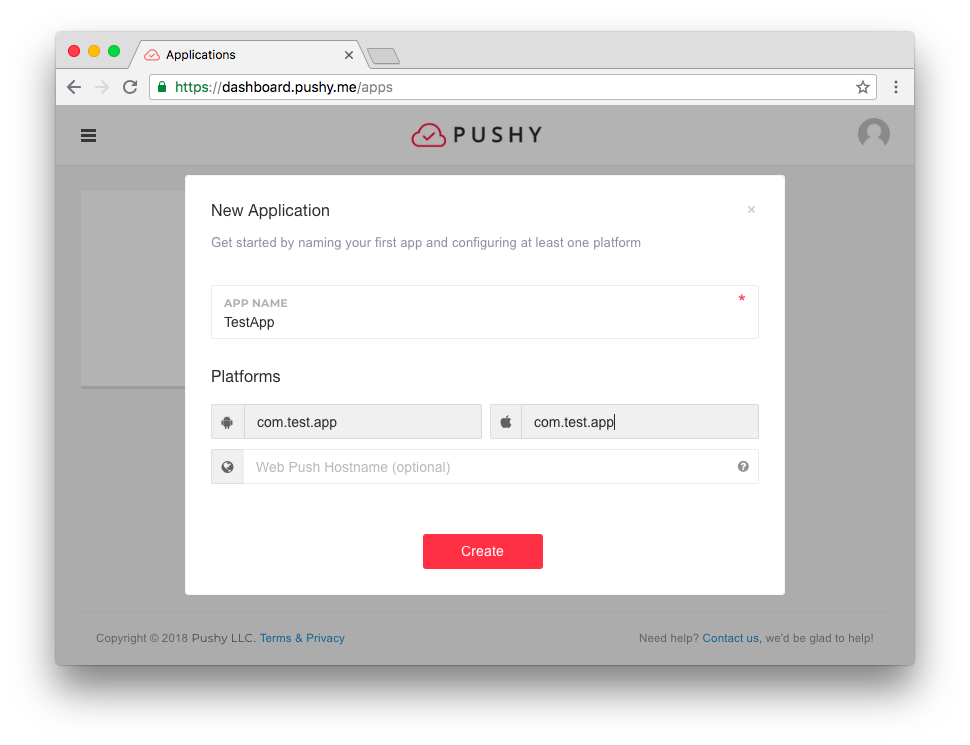
Note: If you have already created an app in the Pushy Dashboard for another platform, simply configure your existing dashboard app with your Android Package Name & iOS Bundle ID in the App Settings tab and proceed to the next step.
Click Create and proceed to the next step.
Install the SDK
Run the following command in the root directory of your project to install the pushy-react-native package from npm:
npm install pushy-react-native --save
Expo: Install the Config Plugin
Note: Skip this step if your React Native project is not an Expo project.
Run the following command in the root directory of your project to install the pushy-expo-plugin from npm:
npx expo install pushy-expo-plugin
You may skip the "Enable Push Capability (iOS)" step below and continue with Configure Expo Router.
Enable Push Capability (iOS)
Enable the Push Notifications
capability manually for your iOS app to register for and receive push notifications.
Open your React Native iOS app in Xcode by running the following command in your project root folder:
open ios/*.xcworkspace
Then, visit the project editor, select the Signing & Capabilities tab, click + Capability and then enable the Push Notifications
capability:
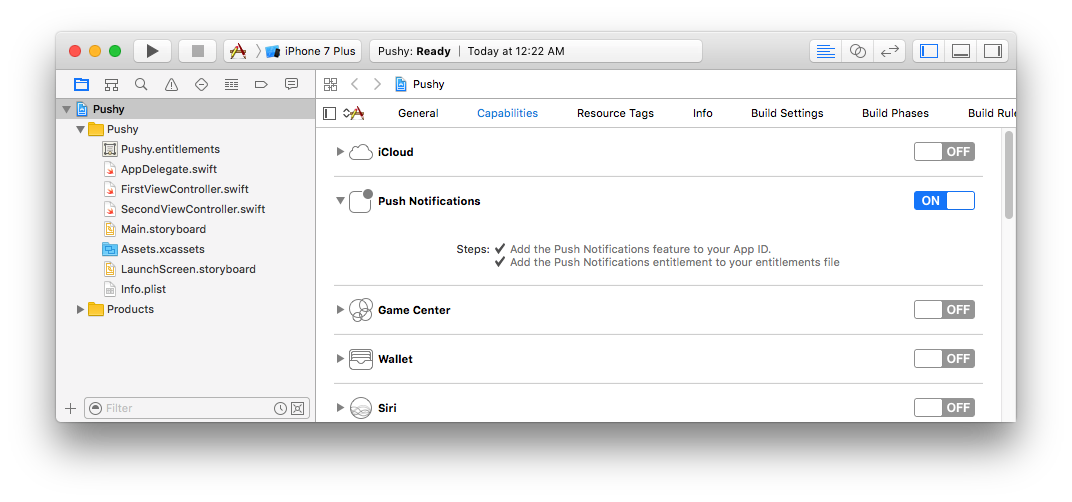
Note: Xcode should display two checkmarks indicating that the capability was successfully enabled.
You may close the Xcode project now.
Configure Expo Router
If your React Native Expo project makes use of expo-router
, please create a file called app/App.tsx
with the following contents:
import 'expo-router/entry'
export default function() {
// Leave empty
}
Then, modify your project's package.json
and find the following line:
"main": "expo-router/entry"
Replace with:
"main": "app/App.tsx",
This is necessary for being able to process push notifications with the app in the background/killed state using HeadlessJS.
Modify App.tsx
Require the package in your App.tsx
file like so:
import Pushy from 'pushy-react-native';
Invoke Pushy.listen()
in your App.tsx
or within another component's lifecycle method to initialize the Pushy SDK:
// Start the Pushy service
Pushy.listen();
Register Devices
Users need to be uniquely identified to receive push notifications.
Every user is assigned a unique device token that you can use to push it at any given time. Once the user has been assigned a device token, it should be stored in your application's backend database.
Add the following code within a component lifecycle method to register the device for push notifications:
// Register the user for push notifications
Pushy.register().then(async deviceToken => {
// Display an alert with device token
alert('Pushy device token: ' + deviceToken);
// Print token to developer console
console.log('Pushy device token: ' + deviceToken);
// Send the token to your backend server via an HTTP GET request
//await fetch('https://your.api.hostname/register/device?token=' + deviceToken);
// Succeeded, optionally do something to alert the user
})
.catch(err => {
// Notify user of failure
alert('Registration failed: ' + err.message);
});
Listen for Notifications
Call the Pushy.setNotificationListener(data => {})
method in your App.tsx
file, right after the import
statements, outside any component lifecycle method declaration:
// Place these lines of code right after the
// import statements in App.tsx as top-level code
Pushy.setNotificationListener(async data => {
// Print notification payload data
console.log('Received notification: ' + JSON.stringify(data));
// Notification title
let notificationTitle = 'MyApp';
// Attempt to extract the "message" property from the payload: {"message":"Hello World!"}
let notificationText = data.message || 'Test notification';
// Android: Displays a system notification
// iOS: Displays an alert dialog
Pushy.notify(notificationTitle, notificationText, data);
// Clear iOS badge count
Pushy.setBadge(0);
});
// Enable in-app notification banners (iOS 10+)
Pushy.toggleInAppBanner(true);
These methods must only be called from within your App.tsx
file, and should not be invoked from within a component lifecycle method, or any other project file.
Note: Please make sure to add the android.permission.VIBRATE
permission declaration within your android/app/src/main/AndroidManifest.xml
if you'd like the notification to vibrate the device.
Feel free to modify this sample code to suit your own needs.
Listen for Notification Click
Call the Pushy.setNotificationClickListener(data => {})
method anywhere in your application to listen for when the user taps your notifications:
// Handle notification tap event
Pushy.setNotificationClickListener(async data => {
// Display basic alert
alert('Notification click: ' + data.message);
// Navigate the user to another page or
// execute other logic on notification click
});
Custom Notification Icon (Android)
Optionally configure a custom notification icon for incoming Android notifications by placing icon file(s) in android/app/src/main/res/drawable-*
and calling:
Pushy.setNotificationIcon('ic_notification');
Please invoke this method after Pushy.listen()
and replace ic_notification
with the resource file name, excluding the extension.
Note: If you don't call this method, or an invalid resource is provided, a generic icon will be used instead.
Parse Notification Data
Any payload data that you send with your push notifications is made available to your app via the data
parameter of your notification listener.
If you were to send a push notification with the following payload:
{"id": 1, "success": true, "message": "Hello World"}
Then you'd be able to retrieve each value from within your notification listener callback like so:
let id = data.id; // number
let success = data.success; // bool
let message = data.message; // string
Note: Unlike GCM / FCM, we do not stringify your payload data, except if you supply JSON objects or arrays.
Subscribe to Topics
Optionally subscribe the user to one or more topics to target multiple users with a shared interest when sending notifications.
Depending on your app's notification criteria, you may be able to leverage topics to simply the process of sending the same notification to multiple users. If your app only sends personalized notifications, skip this step and simply target individual users by their unique device tokens.
Add the following code within a component lifecycle method to subscribe the user to a topic:
// Make sure the user is registered
Pushy.isRegistered().then(isRegistered => {
if (isRegistered) {
// Subscribe the user to a topic
Pushy.subscribe('news').then(() => {
// Subscribe successful
alert('Subscribed to topic successfully');
}).catch(err => {
// Notify user of failure
alert('Subscribe failed: ' + err.message);
});
}
});
Note: Replace news
with your own case-sensitive topic name that matches the following regular expression: [a-zA-Z0-9-_.]{1,100}
.
You can then notify multiple users subscribed to a certain topic by specifying the topic name (prefixed with /topics/
) as the to
parameter in the Send Notifications API.
Setup APNs Authentication (iOS)
Configure the Pushy dashboard with an APNs Auth Key in order to send notifications to your iOS users.
Pushy routes your iOS push notifications through APNs, the Apple Push Notification Service. To send push notifications with APNs, we need to be able to authenticate on behalf of your app. This is achieved by generating an APNs Auth Key and uploading it to the Dashboard.
In the Apple Developer Center, visit the Auth Keys creation page.
- Enter a Key Name.
- Enable Apple Push Notifications service (APNs).
- Click Configure to the right of Apple Push Notifications service (APNs).
- For Environment, make sure to select Sandbox & Production.
- For Key Restriction, make sure to select Team Scoped (All Topics).
Click Save followed by Continue followed
by Register to download the .p8
key file:
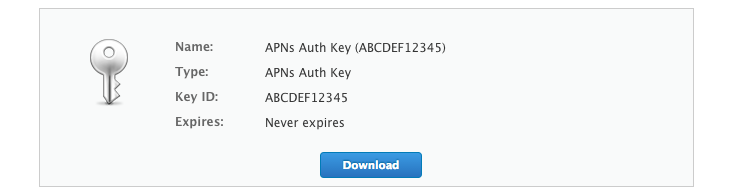
Note: Keep track of the assigned Key ID for the next step.
Visit the Pushy Dashboard -> Click your app -> App Settings -> Configure APNs Auth ().
Fill in the following:
- APNs Auth Key - drag and drop the auth key
.p8
file from the previous step - Key ID - the Key ID assigned to the key you downloaded in the previous step
- Team ID - the Team ID of your Apple Developer Account, as specified in Membership Details
Click Upload to finish setting up APNs authentication for your app.
Note: Avoid revoking your APNs Auth Key in the Apple Developer Center. Otherwise, you will not be able to send notifications with Pushy. If you think your key was compromised, generate a new one in the Apple Developer Center. Then, upload it to the Dashboard. Finally, revoke your old key in the Apple Developer Center.
Select Development Team (Xcode)
Ensure you have selected a Development Team manually for your iOS project to register for and receive push notifications. Open your React Native / Expo iOS app in Xcode by running the following command in your project root folder:
open ios/*.xcworkspace
Then, visit the project editor, select the Signing & Capabilities tab, and ensure your team is selected under the Team dropdown, and that Automatically manage signing is enabled.
Send Test Notification
Run your app, input your device token, and select your app to send a test notification:
Note: You can specify a topic instead of a device token (i.e. /topics/news
). Also, if your app is not automatically detected, please manually copy the Secret API Key from the Dashboard and paste it into the form.
Did you receive the notification? If not, reach out, we'll be glad to help.
Congratulations on implementing Pushy in your React Native / Expo app!
To start sending push notifications to your users, start persisting device tokens in your backend, and invoke the Send Notifications API when you want to send a notification. Follow our step-by-step guide: