PhoneGap Setup
Send push notifications to your PhoneGap app with Pushy.
Create an App
Sign up for Pushy Trial to get started, or log in to your existing account
Note: No credit card needed. Upgrade to the Pro plan when you're ready by visiting the Billing page.
Create an app and fill in the Android Package Name & iOS Bundle ID:
Please ensure the Android Package Name & iOS Bundle ID you enter precisely match the ones configured in your project files, as they are used to automatically link your client apps with the Pushy Dashboard app having the same Android Package Name & iOS Bundle ID.
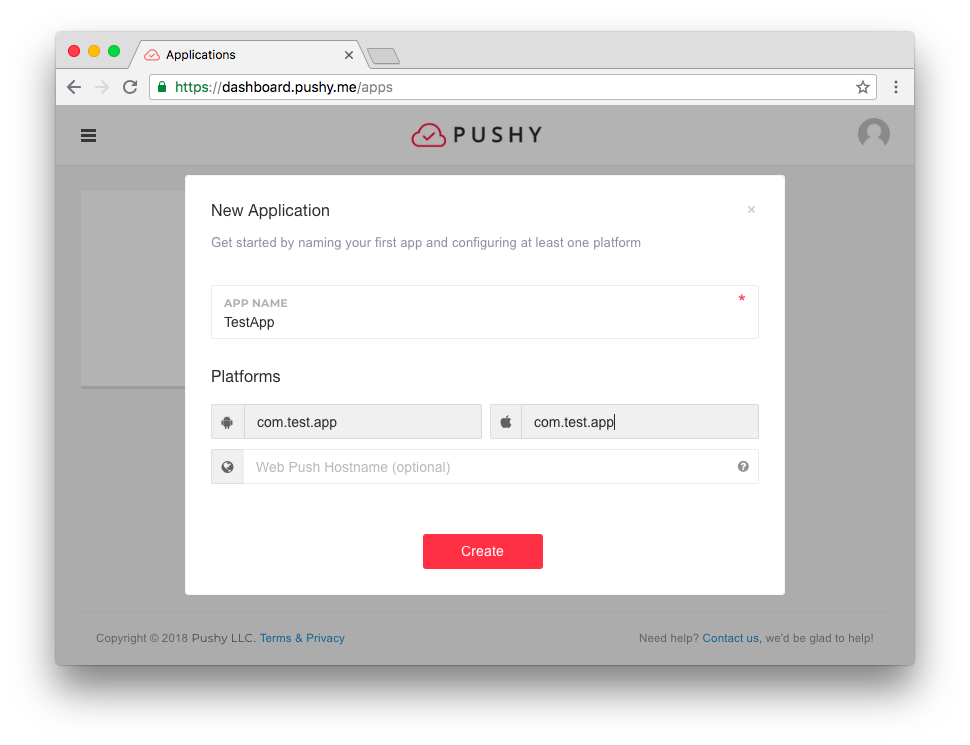
Note: If you have already created an app in the Pushy Dashboard for another platform, simply configure your existing dashboard app with your Android Package Name & iOS Bundle ID in the App Settings tab and proceed to the next step.
Click Create and proceed to the next step.
Get the SDK
Install the latest version of our Cordova SDK by running the following command in the root directory of your project:
phonegap plugin add pushy-cordova
Install Push Receiver (Android)
Install the Android push BroadcastReceiver
plugin by running the following:
phonegap plugin add ./plugins/pushy-cordova/receiver/
Note: This command installs the pushy-cordova-receiver
plugin from your local filesystem to make it easier to modify its implementation later, if needed.
Modify Index File
Invoke Pushy.listen()
in your www/js/index.js
file's onDeviceReady()
method so that Pushy's internal notification listening service will start itself, if necessary:
// Start the Pushy service
Pushy.listen();
Register Devices
Users need to be uniquely identified to receive push notifications.
Every user is assigned a unique device token that you can use to push it at any given time. Once the user has been assigned a device token, it should be stored in your application's backend database.
Add the following code to your application to register the device for push notifications:
// Register the user for push notifications
Pushy.register(function (err, deviceToken) {
// Handle registration errors
if (err) {
return alert(err);
}
// Display an alert with device token
alert('Pushy device token: ' + deviceToken);
// Send the token to your backend server via an HTTP GET request
//await fetch('https://your.api.hostname/register/device?token=' + deviceToken);
// Succeeded, optionally do something to alert the user
});
Listen for Notifications
Call the Pushy.setNotificationListener((data) => {})
method from your application to listen for when incoming push notifications are received:
// Enable in-app notification banners (iOS 10+)
Pushy.toggleInAppBanner(true);
// Listen for push notifications
Pushy.setNotificationListener(function (data) {
// Print notification payload data
console.log('Received notification: ' + JSON.stringify(data));
// Display an alert with the "message" payload value
alert('Received notification: ' + data.message);
// Clear iOS app badge number
Pushy.clearBadge();
});
Feel free to modify this sample code to suit your own needs.
Note: This callback is only invoked if incoming notifications are received while your app is in foreground. Otherwise, this callback will be invoked the next time your app goes to foreground.
Listen for Notification Click
Optionally call the Pushy.setNotificationClickListener((data) => {})
method from your application to listen for when the user taps your notifications:
// Listen for notification click
Pushy.setNotificationClickListener(function (data) {
// Print notification payload data
console.log('Notification click: ' + JSON.stringify(data));
// Display an alert with the "message" payload value
alert('Notification click: ' + data.message);
// Navigate the user to another page or
// execute other logic on notification click
});
Custom Notification Icon (Android)
Optionally configure a custom notification icon for incoming Android notifications by placing icon file(s) in platforms/android/app/src/main/res/drawable-*
and calling:
Pushy.setNotificationIcon('ic_notification');
Please invoke this method after Pushy.listen()
and replace ic_notification
with the resource file name, excluding the extension.
Note: If you don't call this method, or an invalid resource is provided, a generic icon will be used instead.
Parse Notification Data
Any payload data that you send with your push notifications is made available to your app via the data
parameter of your notification listener.
If you were to send a push notification with the following payload:
{"id": 1, "success": true, "message": "Hello World"}
Then you'd be able to retrieve each value from within your notification listener callback like so:
var id = data.id; // number
var success = data.success; // bool
var message = data.message; // string
Note: Unlike GCM / FCM, we do not stringify your payload data, except if you supply JSON objects or arrays.
Subscribe to Topics
Optionally subscribe the user to one or more topics to target multiple users with a shared interest when sending notifications.
Depending on your app's notification criteria, you may be able to leverage topics to simply the process of sending the same notification to multiple users. If your app only sends personalized notifications, skip this step and simply target individual users by their unique device tokens.
Add the following code to your application to subscribe the user to a topic:
// Make sure the user is registered
Pushy.isRegistered(function (isRegistered) {
if (isRegistered) {
// Subscribe the user to a topic
Pushy.subscribe('news', function (err) {
// Handle errors
if (err) {
return alert(err);
}
// Subscribe successful
alert('Subscribed to topic successfully');
});
}
});
Note: Replace news
with your own case-sensitive topic name that matches the following regular expression: [a-zA-Z0-9-_.]{1,100}
.
You can then notify multiple users subscribed to a certain topic by specifying the topic name (prefixed with /topics/
) as the to
parameter in the Send Notifications API.
Modify the Android Push Receiver (Optional)
Optionally modify the default Android push BroadcastReceiver
implementation to handle incoming notifications differently.
The built-in push receiver emits a system notification with your app name and icon, and extracts the message from the {"message": "..."}
JSON data payload value. If this behavior is sufficient for your app, you may skip this step.
Modify the PushReceiver.java
file in the following path:
plugins/pushy-cordova/receiver/src/android/PushReceiver.java
Note: Do not accidentally modify plugins/pushy-cordova-receiver/src/android/PushReceiver.java
or platforms/android/src/me/pushy/sdk/cordova/PushReceiver.java
as your modifications will be lost when you reinstall the plugin.
When you are done modifying the PushReceiver.java
implementation, reinstall the plugin locally for changes to take effect by running the following commands in your root project directory:
phonegap plugin rm pushy-cordova-receiver
phonegap plugin add ./plugins/pushy-cordova/receiver/
Setup APNs Authentication (iOS)
Configure the Pushy dashboard with an APNs Auth Key in order to send notifications to your iOS users.
Pushy routes your iOS push notifications through APNs, the Apple Push Notification Service. To send push notifications with APNs, we need to be able to authenticate on behalf of your app. This is achieved by generating an APNs Auth Key and uploading it to the Dashboard.
In the Apple Developer Center, visit the Auth Keys creation page.
- Enter a Key Name.
- Enable Apple Push Notifications service (APNs).
- Click Configure to the right of Apple Push Notifications service (APNs).
- For Environment, make sure to select Sandbox & Production.
- For Key Restriction, make sure to select Team Scoped (All Topics).
Click Save followed by Continue followed
by Register to download the .p8
key file:
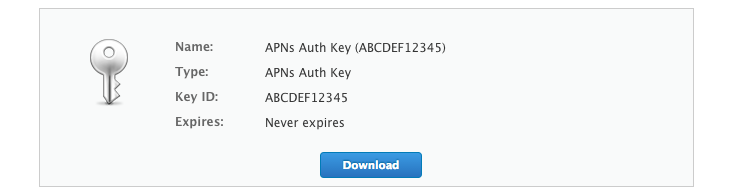
Note: Keep track of the assigned Key ID for the next step.
Visit the Pushy Dashboard -> Click your app -> App Settings -> Configure APNs Auth ().
Fill in the following:
- APNs Auth Key - drag and drop the auth key
.p8
file from the previous step - Key ID - the Key ID assigned to the key you downloaded in the previous step
- Team ID - the Team ID of your Apple Developer Account, as specified in Membership Details
Click Upload to finish setting up APNs authentication for your app.
Note: Avoid revoking your APNs Auth Key in the Apple Developer Center. Otherwise, you will not be able to send notifications with Pushy. If you think your key was compromised, generate a new one in the Apple Developer Center. Then, upload it to the Dashboard. Finally, revoke your old key in the Apple Developer Center.
Build and Test
Run your app on each platform to make sure everything works as expected:
phonegap run platform
Note: Replace platform
with both ios
and android
to test both integrations, one at a time.
Send Test Notification
Input your device token and select your app to send a test push notification:
Note: You can specify a topic instead of a device token (i.e. /topics/news
). Also, if your app is not automatically detected, please manually copy the Secret API Key from the Dashboard and paste it into the form.
Did you receive the notification? If not, reach out, we'll be glad to help.
Congratulations on implementing Pushy in your PhoneGap app!
To start sending push notifications to your users, start persisting device tokens in your backend, and invoke the Send Notifications API when you want to send a notification. Follow our step-by-step guide: